View previous topic :: View next topic |
Author |
Message |
Sabre
Joined: 14 Sep 2007 Posts: 13

|
Posted: Thu Feb 02, 2023 3:01 pm Post subject: Communication with VEML6040 color sensor (problem solved) |
|
|
I'm trying to write a program to communicate with a VEML6040 color sensor. I am not able to read the color values from the registers of this sensor. Is my code correct? Specifically initializing the sensor?
Code: | $Regfile="m328pdef.dat"
$Crystal=16000000
$hwstack=40
$swstack=16
$framesize=32
$lib "i2c_twi.lbx" 'Hardware I2C
config Scl = Portc.5
Config Sda = Portc.4
Config Twi = 400000
Config Lcdpin = Pin , Db4 = Portb.2 , Db5 = Portb.3 , Db6 = Portb.4 , Db7 = Portb.5 , E = Portb.0 , Rs = Portb.1
Config Lcd = 16 * 2
Declare Sub Veml6040_init()
Declare Sub Read_rgb_data()
Const Device_address = &H10
Dim R_color As Word
Dim G_color As Word
Dim B_color As Word
Dim R_high As Byte
Dim R_low As Byte
Dim G_high As Byte
Dim G_low As Byte
Dim B_high As Byte
Dim B_low As Byte
const R_ADR = &H08
const G_ADR = &H09
const B_ADR = &H0A
const W_ADR = &H0B
Veml6040_init
Do
Read_rgb_data
Cls
Locate 1 , 1
Lcd "R: " ; R_color
Locate 2 , 1
Lcd "G: " ; G_color
Locate 1 , 9
Lcd "B: " ; B_color
Waitms 200
Loop
End
Sub Veml6040_init()
I2cstart
I2cwbyte Device_address
I2cwbyte &H00
I2cwbyte &H20
I2cwbyte &H00
I2cstop
End Sub
Sub Read_rgb_data()
I2cstart
I2cwbyte Device_address
I2cstart
I2cwbyte r_adr
I2crbyte R_high, Ack
I2crbyte R_low, nack
I2cstop
I2cstart
I2cwbyte g_adr
I2crbyte G_high, Ack
I2crbyte G_low, nack
I2cstop
I2cstart
I2cwbyte b_adr
I2crbyte B_high, Ack
I2crbyte B_low, Nack
I2cstop
R_color = R_high * 256
R_color=R_color + R_low
G_color = G_high * 256
G_color=G_color + G_low
B_color = B_high * 256
B_color=B_color+ B_low
End Sub |
(BASCOM-AVR version : 2.0.8.5 )
Last edited by Sabre on Thu Feb 02, 2023 7:17 pm; edited 2 times in total |
|
Back to top |
|
 |
programmista123
Joined: 31 Jan 2018 Posts: 191

|
Posted: Thu Feb 02, 2023 4:14 pm Post subject: |
|
|
Hello,
Sub Read_rgb_data() is not correct.
Code: |
I2cstart
I2cwbyte Device_address
I2cstart
I2cwbyte r_adr
I2crbyte R_high, Ack
I2crbyte R_low, nack
I2cstop
|
You need to have:
1. I2C start
2. Command Code
3. Slave address
4. Data
+ I2CREPSTART instead of I2cstart again.
So, for example R read should looks like:
Code: |
I2cstart 'Start
I2cwbyte Device_address 'Address
I2cwbyte r_adr
I2CREPSTART
I2cwbyte Device_address
I2crbyte R_high, Ack
I2crbyte R_low, nack
I2cstop
|
Regards,
Przemek |
|
Back to top |
|
 |
Sabre
Joined: 14 Sep 2007 Posts: 13

|
Posted: Thu Feb 02, 2023 4:22 pm Post subject: |
|
|
programmista123 wrote: | Hello,
Sub Read_rgb_data() is not correct.
Code: |
I2cstart
I2cwbyte Device_address
I2cstart
I2cwbyte r_adr
I2crbyte R_high, Ack
I2crbyte R_low, nack
I2cstop
|
You need to have:
1. I2C start
2. Command Code
3. Slave address
4. Data
+ I2CREPSTART instead of I2cstart again.
So, for example R read should looks like:
Code: |
I2cstart 'Start
I2cwbyte Device_address 'Address
I2cwbyte r_adr
I2CREPSTART
I2cwbyte Device_address
I2crbyte R_high, Ack
I2crbyte R_low, nack
I2cstop
|
Regards,
Przemek |
Hi, thanks for reply, but I have a question, why is the address of the device sent 2 times in your example code? |
|
Back to top |
|
 |
programmista123
Joined: 31 Jan 2018 Posts: 191

|
Posted: Thu Feb 02, 2023 4:26 pm Post subject: |
|
|
Datasheet
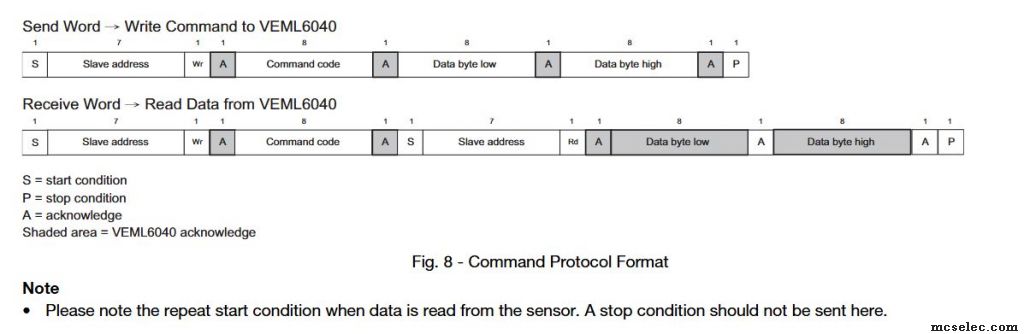 |
|
Back to top |
|
 |
Sabre
Joined: 14 Sep 2007 Posts: 13

|
Posted: Thu Feb 02, 2023 4:33 pm Post subject: |
|
|
This change in the code resulted in the display showing 4112 for each color. This value does not change depending on the color of the substrate over which the sensor is placed.
Maybe there is something wrong with the initialization of the sensor?
I also changed the order of receiving bytes from the sensor according to the documentation, the first is byte low, the second is byte high. It didn't change anything.
Code: | I2cstart 'Start
I2cwbyte Device_address 'Address
I2cwbyte r_adr
I2CREPSTART
I2cwbyte Device_address
I2crbyte R_low, Ack
I2crbyte R_high, nack
I2cstop |
|
|
Back to top |
|
 |
MWS
Joined: 22 Aug 2009 Posts: 2335

|
Posted: Thu Feb 02, 2023 5:50 pm Post subject: |
|
|
programmista123 wrote: | Datasheet |
You both need to read the important parts:
Quote: | VEML6040 uses 10h slave address for 7-bit I2C addressing protocol. |
|
|
Back to top |
|
 |
Sabre
Joined: 14 Sep 2007 Posts: 13

|
Posted: Thu Feb 02, 2023 6:25 pm Post subject: |
|
|
Ok, what does this change in communication or my specific example? Should I shift the address to the left and add a 0 or a 1 to the lowest bit? I thought Bascom supported 7 bit addressing.
I changed the address for writing data to &H20 and reading to &H21 and the program started working.
MWS, programmista123 thanks for your help and pointing me in the right direction .
Here is the working code:
Code: | $Regfile="m328pdef.dat"
$Crystal=16000000
$hwstack=40
$swstack=16
$framesize=32
$lib "i2c_twi.lbx" 'Hardware I2C
config Scl = Portc.5
Config Sda = Portc.4
Config Twi = 100000
Config Lcdpin = Pin , Db4 = Portb.2 , Db5 = Portb.3 , Db6 = Portb.4 , Db7 = Portb.5 , E = Portb.0 , Rs = Portb.1
Config Lcd = 16 * 2
Declare Sub Veml6040_init()
Declare Sub Read_rgb_data()
Dim R_color As Word
Dim G_color As Word
Dim B_color As Word
Dim R_high As Byte
Dim R_low As Byte
Dim G_high As Byte
Dim G_low As Byte
Dim B_high As Byte
Dim B_low As Byte
Const Device_write_address = &H20
Const Device_read_address = &H21
const R_ADR = &H08
const G_ADR = &H09
const B_ADR = &H0A
const W_ADR = &H0B
Veml6040_init
Do
Read_rgb_data
Cls
Locate 1 , 1
Lcd "R: " ; R_color
Locate 2 , 1
Lcd "G: " ; G_color
Locate 1 , 9
Lcd "B: " ; B_color
Waitms 200
Loop
End
Sub Veml6040_init()
I2cstart
I2cwbyte Device_write_address
I2cwbyte &H00
I2cwbyte &H20
I2cwbyte &H00
' I2cwbyte &H00
I2cstop
End Sub
Sub Read_rgb_data()
I2cstart 'Start
I2cwbyte Device_write_address 'Address
I2cwbyte r_adr
I2CREPSTART
I2cwbyte Device_read_address
I2crbyte R_low, Ack
I2crbyte R_high, nack
I2cstop
I2cstart
I2cwbyte Device_write_address
I2cwbyte g_adr
I2CREPSTART
I2cwbyte Device_read_address
I2crbyte G_low, Ack
I2crbyte G_high, nack
I2cstop
I2cstart
I2cwbyte Device_write_address
I2cwbyte b_adr
I2CREPSTART
I2cwbyte Device_read_address
I2crbyte B_low, Ack
I2crbyte B_high, Nack
I2cstop
R_color = R_high * 256
R_color=R_color + R_low
G_color = G_high * 256
G_color=G_color + G_low
B_color = B_high * 256
B_color=B_color+ B_low
End Sub |
|
|
Back to top |
|
 |
MWS
Joined: 22 Aug 2009 Posts: 2335

|
Posted: Thu Feb 02, 2023 7:07 pm Post subject: |
|
|
Ok, good. |
|
Back to top |
|
 |
Sabre
Joined: 14 Sep 2007 Posts: 13

|
Posted: Thu Feb 02, 2023 7:15 pm Post subject: |
|
|
MWS, I dealt with the addresses based on the table from the documentation (after shifting &H10 to left I added to the address 0 when writing the configuration and 1 when reading data) and published the working code, read the entire post above. |
|
Back to top |
|
 |
MWS
Joined: 22 Aug 2009 Posts: 2335

|
Posted: Thu Feb 02, 2023 7:26 pm Post subject: |
|
|
I did notice that, as you can see from the change of my former reply. |
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You cannot download files in this forum
|
|